Scene Magnitude and adding new primitives
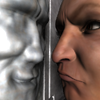
Hi all,
I am trying to write a command that adds a primitive to a scene. The problem is that the primitive does not scale to the Magnitude of the scene when I insert it into the instance tree. I have found that I have to multiply everything by the scene->GetMagnitude() value to get it to be created at the right scale. Here is my function:
boolean TestCommand::Do()
{
TMCCountedPtr masterObject;
MCIID riid;
TMCCountedPtr params;
gShell3DUtilities->CreatePrimitiveByID(kCubePrimitiveID, &masterObject;, IID_IShParameterComponent, (void**)¶ms;);
TMCCountedPtr instance;
gComponentUtilities->CoCreateInstance(CLSID_StandardInstance, NULL, MC_CLSCTX_INPROC_SERVER, IID_I3DShInstance, (void **) &instance;);
instance->Set3DObject(masterObject);
TMCString31 name;
params->GetClassNameA(name);
instance->GetTreeElement()->SetName(name);
real32 value;
params->GetParameter('scal', &value;);
value *= fScene->GetMagnitude();
params->SetParameter('scal', &value;);
instance->GetTreeElement()->SetMasterGroup(fMasterGroup);
instance->GetTreeElement()->CenterHotPointOnElement();
fTree->InsertLast(instance->GetTreeElement());
TTreeTransform globalT;
globalT.ConvertMode(TTreeTransform::kCarrara);
globalT.SetUniformScaling(fScene->GetMagnitude());
globalT.SetOffset(TVector3(0, 0, value / 2.0F));
instance->GetTreeElement()->SetGlobalTreeTransform(globalT);
return true;
}
Notice all the places I need to apply the scene Magnitude to get the cube to be inserted at the right scale. I was just wondering if there was a better way to do this. I am hoping for something that does not require me to crack open every primitive to adjust its size.
Comments
You may have noticed you are getting odd results because you are not adding the masterObject to the scene. After you create the masterObject do:
Then you can set the name and create the instance.
You don't need to set the transform.
You can continue to use the params->SetParameter or you can do the following: